Question
How to do python PWM?
Answer
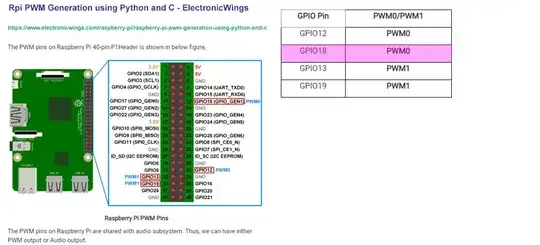
Your program is not very clearly commented, You might like to compare and contrast a similar python PWM segment, in my debugged, complete, copy-paste-run program.
# *** GPIO PWM Mode Setup and PWM Output ***
def setGpioPinPwmMode(gpioPinNum, frequency):
pwmPinObject = GPIO.PWM(gpioPinNum, frequency)
return pwmPinObject
def pwmPinChangeFrequency(pwmPinObject, frequency):
pwmPinObject.ChangeFrequency(frequency)
return
def pwmPinChangeDutyCycle(pwmPinObject, dutyCycle):
pwmPinObject.ChangeDutyCycle(dutyCycle)
return
def pwmPinStart(pwmPinObject):
initDutyCycle = 50
pwmPinObject.start(initDutyCycle)
return
def pwmPinStop(pwmPinObject):
pwmPinObject.stop()
return
Notes
My program is written for controlling a toy servo. But you don't need a servo to test. The main point is to understand how to set, start, and stop the PWM pin, which is connected to a servo or a LED. If you use a LED instead of a servo, you should see the LED goes very dim if duty cycle is very small, and full bright if big duty cycle.
Rpi python is running a toy servo at 50Hz PWM. It might go crazy if you ask it to run so ridiculously low a frequency of 0.5Hz. Rpi might complain that 0.5 is not a frequency value!
If you set frequency higher than 25Hz, we stupid human eyes are not fast to catch, so you see that in my servo tests I need to use a oscilloscope to watch the PWM signal blinking in "slow motion".
References
(1) How can Rpi move a Servo motor using a GPIO pin in PWM mode?, RpiSE 2019may11, Viewed 2k times
Appendices
Appendix A - Rpi python program to PWM run a servo (or blink a LED ridiculously fast)
# Servo_test32 tlfong01 2019may12hkt1506 ***
# Raspbian stretch 2019apr08, Python 3.5.3
# Reference: How can Rpi move a Servo motor using a GPIO pin in PWM mode?, RpiSE 2019may11, Viewed 2k times
# https://raspberrypi.stackexchange.com/questions/98467/how-can-rpi-move-a-servo-motor-using-a-gpio-pin-in-pwm-mode
import RPi.GPIO as GPIO
from time import sleep
# *** GPIO Housekeeping Functions ***
def setupGpio():
GPIO.setmode(GPIO.BCM)
GPIO.setwarnings(False)
return
def cleanupGpio():
GPIO.cleanup()
return
# *** GPIO Input/Output Mode Setup and High/Low Level Output ***
def setGpioPinLowLevel(gpioPinNum):
lowLevel = 0
GPIO.output(gpioPinNum, lowLevel)
return
def setGpioPinHighLevel(gpioPinNum):
highLevel = 1
GPIO.output(gpioPinNum, highLevel)
return
def setGpioPinOutputMode(gpioPinNum):
GPIO.setup(gpioPinNum, GPIO.OUT)
setGpioPinLowLevel(gpioPinNum)
return
# *** GPIO PWM Mode Setup and PWM Output ***
def setGpioPinPwmMode(gpioPinNum, frequency):
pwmPinObject = GPIO.PWM(gpioPinNum, frequency)
return pwmPinObject
def pwmPinChangeFrequency(pwmPinObject, frequency):
pwmPinObject.ChangeFrequency(frequency)
return
def pwmPinChangeDutyCycle(pwmPinObject, dutyCycle):
pwmPinObject.ChangeDutyCycle(dutyCycle)
return
def pwmPinStart(pwmPinObject):
initDutyCycle = 50
pwmPinObject.start(initDutyCycle)
return
def pwmPinStop(pwmPinObject):
pwmPinObject.stop()
return
# *** Test Functions ***
def setHighLevelGpioPin18():
print(' Begin setHighLevelGpioPin18, ...')
gpioPinNum = 18
sleepSeconds = 2
setupGpio()
setGpioPinOutputMode(gpioPinNum)
setGpioPinHighLevel(gpioPinNum)
sleep(sleepSeconds)
cleanupGpio()
print(' End setHighLevelGpioPin18, ...\r\n')
return
def setPwmModeGpioPin18():
print(' Begin setPwmModeGpioPin18, ...')
gpioPinNum = 18
sleepSeconds = 10
frequency = 1000
dutyCycle = 50
setupGpio()
setGpioPinOutputMode(gpioPinNum)
pwmPinObject = setGpioPinPwmMode(gpioPinNum, frequency)
pwmPinStart(pwmPinObject)
pwmPinChangeFrequency(pwmPinObject, frequency)
pwmPinChangeDutyCycle(pwmPinObject, dutyCycle)
sleep(sleepSeconds)
pwmPinObject.stop()
cleanupGpio()
print(' End setPwmModeGpioPin18, ...\r\n')
return
# *** Main ***
print('Begin testing, ...\r\n')
setHighLevelGpioPin18()
setPwmModeGpioPin18()
print('End testing.')
# *** End of program ***
'''
Sample Output - 2019may12hkt1319
>>>
RESTART: /home/pi/Python Programs/Python_Programs/test1198/servo_test31_2019may1201.py
Begin testing, ...
Begin setHighLevelGpioPin18, ...
End setHighLevelGpioPin18, ...
Begin setPwmModeGpioPin18, ...
End setPwmModeGpioPin18, ...
End testing.
>>>
>>>
'''