Question
Run [bash/python] automatically at startup, timestamp is wrong, ...
Answer
Or you can try the python datetime thing.
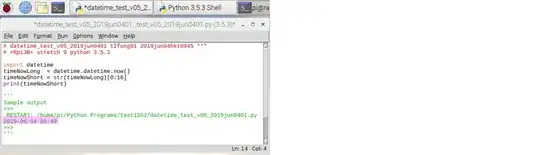
Update 2019jun05hkt2124
The OP says the following:
It works fine if I run the bash script manually through the command
line, but if I allow it to run automatically at startup, the
timestamps for files and archives are completely wrong. I'm not sure
where to start with troubleshooting this issue.
I apologize that I suggested a solution but did not "troubleshoot" or explain why the OP gets the wrong time stamp.
The root cause is that linux does not update the user clock immediately after booting, and its reason is that it has too many higher priority (and less time consuming than getting network time) things to do (in the background) after booting. In other words, it is an engineering trade off and user experience/satisfaction vs system performance.
This is a quick and dirty update. Perhaps I can try a better explanation later.
Update 2019jun06hkt0857
Ideally, linux should keep a record (with the user's authorization) of what the unique user has been doing in the past. Say, if Rpi discovers the angry user, ie, me, hits the little real time clock icon angry (many times in 0.1 second), it knows I want network time update ASAP (of course also checking my Rpi is connected online every time, otherwise it is a waste of time).
Actually MS has been doing research in the past 20+ years for their UI/UE, to make their booting as fast as possible. They from time to time invite users to join their real time online survey observing and collecting statistics on what the gereral/average users do, immediately after booting, and after which job, etc, ... I always agree on their invitation as a volunteer, for the benefit of my user pleasure, and general user's pleasure. Below is an article I read yesterday. Of course you can easily google for many more such articles on UI/UE things. In the past 20+ years or so, I have been on alert on many enggr computing thing, including "Code Project Insider Developer News" where this boot user survey article is coming from.
Microsoft wants to know how you feel about Windows 10’s Start menu - Code Project Insider Developer News Darren Allan 2019jun06
Update 2019jun07hkt2226
pi-wake-on-rtc - bablokb 2018mar09
Introduction
This project implements a wake-on-rtc solution for the Raspberry Pi
and other small computers based on the DS3231 real-time-clock.
Overview
The solution consists of two parts: a circuit doing the actual power
switching and secondly some programs to control the rtc.
The circuit reacts to three events:
a push of the power-on button: this will switch power on and boot the
pi
an alarm of the rtc: this will also turn power on
a gpio-interrupt from the pi after shutdown: this will switch off
power
The software-part takes care to program the alarm of the rtc before shutdown, so the system will boot at the requested time.