Question
Face tracking using laptop usb cam, opencv and dlib
Face motion signal from laptop to Rpi to use GPIO to move servo motors.
Is this config OK?
Answer
I think it is a good use of laptop and Rpi. To know more about how to
use Rpi's PWM GPIO pins to move servo motors, you might like to read
my answer in the following post.
Using Rpi PWM GPIO pin to move servo motors
Swing Servo Youtube - tlfong01 2019may1201
And since the more powerful Rpi4 is coming soon, it is time to plan doing both jobs in Rpi4, saving your laptop for other R&D work.
Update 2019jun07hkt1241
The toy servo I used above as an example is not a good choice, because its positioning is not that precise and stable, not to mention is it is slow, and span limited (about 180 degrees), and has dead bands, ... For more advanced applications, I would recommend cheapy stepping motors and BLDC (BrushLess Direct Current) motors (with and without Hall effect quadrature position encoders) (references to add later). But for rapid prototyping, toy servos are very good。)
References
Pictures showing how Rpi PWM GPIO can move servo motors
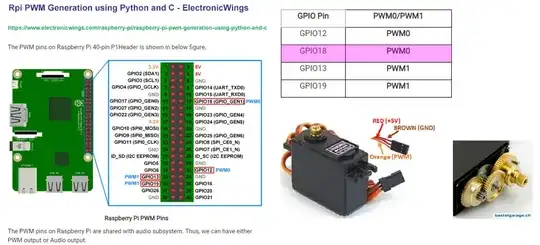
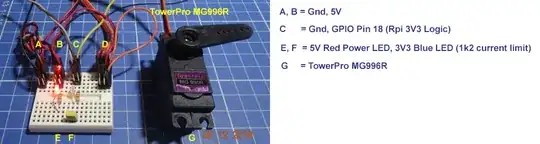
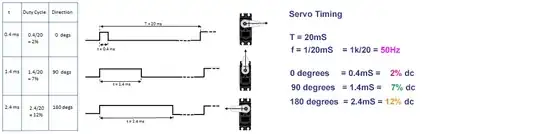

Demo Programs
(1) Demo program
Fully debugged python programs (1) to move motor and (2) display PWM signal, with sample outputs
# Servo_test32 tlfong01 2019may12hkt1506 ***
# Raspbian stretch 2019apr08, Python 3.5.3
import RPi.GPIO as GPIO
from time import sleep
# *** GPIO Housekeeping Functions ***
def setupGpio():
GPIO.setmode(GPIO.BCM)
GPIO.setwarnings(False)
return
def cleanupGpio():
GPIO.cleanup()
return
# *** GPIO Input/Output Mode Setup and High/Low Level Output ***
def setGpioPinLowLevel(gpioPinNum):
lowLevel = 0
GPIO.output(gpioPinNum, lowLevel)
return
def setGpioPinHighLevel(gpioPinNum):
highLevel = 1
GPIO.output(gpioPinNum, highLevel)
return
def setGpioPinOutputMode(gpioPinNum):
GPIO.setup(gpioPinNum, GPIO.OUT)
setGpioPinLowLevel(gpioPinNum)
return
# *** GPIO PWM Mode Setup and PWM Output ***
def setGpioPinPwmMode(gpioPinNum, frequency):
pwmPinObject = GPIO.PWM(gpioPinNum, frequency)
return pwmPinObject
def pwmPinChangeFrequency(pwmPinObject, frequency):
pwmPinObject.ChangeFrequency(frequency)
return
def pwmPinChangeDutyCycle(pwmPinObject, dutyCycle):
pwmPinObject.ChangeDutyCycle(dutyCycle)
return
def pwmPinStart(pwmPinObject):
initDutyCycle = 50
pwmPinObject.start(initDutyCycle)
return
def pwmPinStop(pwmPinObject):
pwmPinObject.stop()
return
# *** Test Functions ***
def setHighLevelGpioPin18():
print(' Begin setHighLevelGpioPin18, ...')
gpioPinNum = 18
sleepSeconds = 2
setupGpio()
setGpioPinOutputMode(gpioPinNum)
setGpioPinHighLevel(gpioPinNum)
sleep(sleepSeconds)
cleanupGpio()
print(' End setHighLevelGpioPin18, ...\r\n')
return
def setPwmModeGpioPin18():
print(' Begin setPwmModeGpioPin18, ...')
gpioPinNum = 18
sleepSeconds = 10
frequency = 1000
dutyCycle = 50
setupGpio()
setGpioPinOutputMode(gpioPinNum)
pwmPinObject = setGpioPinPwmMode(gpioPinNum, frequency)
pwmPinStart(pwmPinObject)
pwmPinChangeFrequency(pwmPinObject, frequency)
pwmPinChangeDutyCycle(pwmPinObject, dutyCycle)
sleep(sleepSeconds)
pwmPinObject.stop()
cleanupGpio()
print(' End setPwmModeGpioPin18, ...\r\n')
return
# *** Main ***
print('Begin testing, ...\r\n')
setHighLevelGpioPin18()
setPwmModeGpioPin18()
print('End testing.')
# *** End of program ***
'''
Sample Output - 2019may12hkt1319
>>>
RESTART: /home/pi/Python Programs/Python_Programs/test1198/servo_test31_2019may1201.py
Begin testing, ...
Begin setHighLevelGpioPin18, ...
End setHighLevelGpioPin18, ...
Begin setPwmModeGpioPin18, ...
End setPwmModeGpioPin18, ...
End testing.
>>>
>>>
'''
(2) Program to display PWM wavefrom on scope
To display PWM waveform
def servoPwmBasicTimingTestGpioPin18():
print(' Begin servoPwmBasicTimingTestGpioPin18, ...')
gpioPinNum = 18
sleepSeconds = 120
frequency = 50
dutyCycle = 7
setupGpio()
setGpioPinOutputMode(gpioPinNum)
pwmPinObject = setGpioPinPwmMode(gpioPinNum, frequency)
pwmPinStart(pwmPinObject)
pwmPinChangeFrequency(pwmPinObject, frequency)
pwmPinChangeDutyCycle(pwmPinObject, dutyCycle)
sleep(sleepSeconds)
pwmPinObject.stop()
cleanupGpio()
print(' End servoPwmBasicTimingTestGpioPin18, ...\r\n')
return
References
(1) Raspberry Pi Servo Motor control - Rpi Tutorials
(2) Servo MG996R Datasheet - TowerPro
(3) Python (RPi.GPIO) API - SparkFun
(4) Using PWM in RPi.GPIO - SourceForge
(5) Raspberry Pi PWM Generation using Python and C - ElectronicWing
(6) Servo Tutorial - Lady Ada
(7) PWM Tutorial - Lady Ada
(8) UniPolar/Bipolar Stepping Motor 28byj48 Using L297D
(10) TowerPro SG5010 360 Degrees Servo
(11) Servo Basics and Control Function Discussion